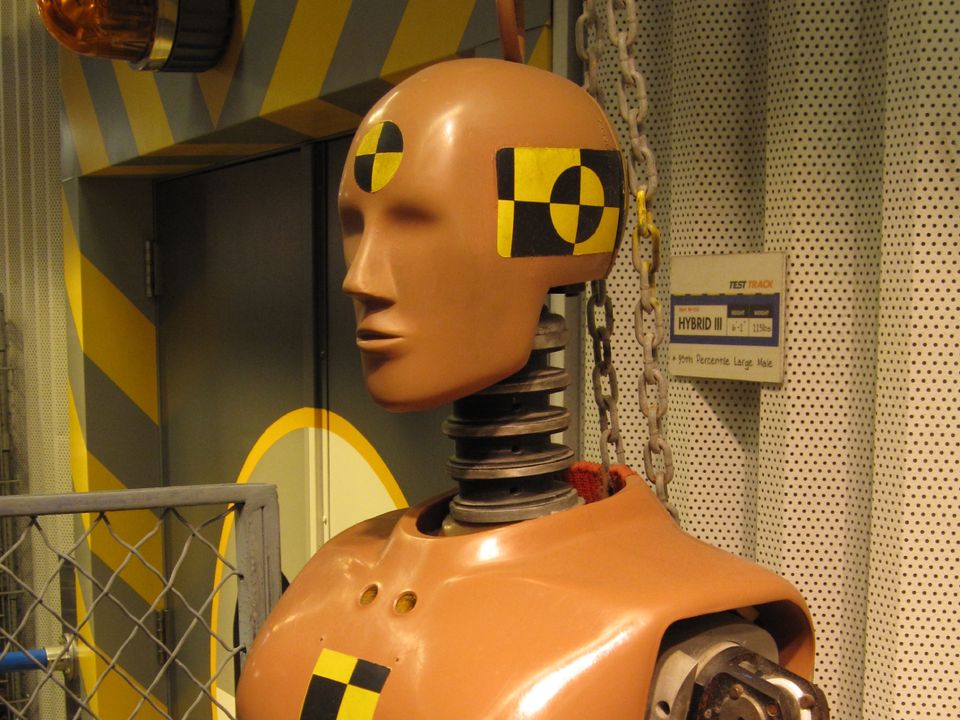
Back in the day, my JS projects were small and self-contained. Nowadays, in my professional work, majority, if not all of front-end applications I am working on are connected to multiple back-end services for variety of reasons. It gives me a freedom of not caring of the back-end, as long as we have defined a contract, and proceeding happily with what actually matters, i.e. colors and animations. But it also gave ma some headache, when I wanted to have pleasant, convenient coding environment on my localhost, and not have all of these weird back-end stuff running on my local machine as well. Here's how I worked it out with both Webpack Dev Server and Express.
I've found two ways of dealing with HTTP requests coming out of my front-end, so that I can handle them freely, without major changes to development version of my application: mocking and proxying. Both are based on intercepting these requests on my localhost level, and handling them manually. However, they are handled differently, and they have their own pros, cons, and ideal case, in which you want to use them.
There is some code in this post, displaying how you can mock or proxy this problem out of your way. There is also some more code on this subject on my github, in code examples section of my blog repository. You'll find a small setup there, which showcases all ideas I'm bragging about in this post. There is an application with a button, that sends HTTP request with fetch; local server, that you can proxy your requests to; and four examples, each demonstrating different solution to this problem. Check it out!
Mocking
With mocked HTTP request response, you don't even call any back-end at all. Request is intercepted, and mocked - manually defined by you - response is served by your localhost. It's useful for most cases, because it gets the job done easily. It's particularly useful, when your back-end is just slow, or there is a lot of data to transfer, or a lot of calls - you just don't transfer any data over the wire, and there is no delay in your development. Downside of this solution is that you never see actual response that is coming from the service. You may end up surprised by totally different data model provided by back-end, or by any other difference between your assumption stated in your mock and actual response.
Webpack Dev Server
proxy
is an option in configuration of Webpack Dev Server, which allows you to define proxy for any HTTP request that comes out of your localhost. You can also define specific bypass in it, with conditions as detailed as you want. In my example, I'm intercepting all calls to /api
URL, and respond with mocked JS object instead.
devServer: {
// ...
proxy: {
'/api': {
bypass: (request, response) => {
if (request.url.substr(-4) === '/api') {
response.send({ data: 'mocked data from webpack.devserver.mock.config.js' })
}
},
},
},
},
(content of the whole script available here)
Express
Contrary to Webpeck Dev Server, mocking a response in Express doesn't feel like hacking. It's very straightforward, almost like this framework was created specifically to perform tasks like this (?!).
const app = express();
// ...
app.get('/api', (request, response) => {
response.json({ data: 'mocked data from express.mock.js' });
});
(content of the whole script available here)
Proxying
With proxied HTTP request, you call actual back-end service. Request is only proxied - redirected to it's true destination, and potentially modified a bit. It's usually preferred for when you are fine with getting real data from real service, even at a cost of waiting some additional time with each visit to your localhost, but your back-end is on different host than your local, which is usually the case. Cons? If you can afford it time- and resources-wise, there are none, in my experience.
Webpack Dev Server
Proxy with Webpack Dev Server can be achieved using proxy
configuration option, this time more like it was originally intended. target
parameter describes destination to which certain call should be redirected. If pathRewrite
parameter is not provided, /api
will be concatenated with the target, which is not what I wanted to achieve here (hence I've defined this parameter), but you may find it useful.
devServer: {
// ...
proxy: {
'/api': {
target: 'http://localhost:3001/get-secret-data',
pathRewrite: { '^/api': '' },
},
},
},
(content of the whole script available here)
Express
In Express, it's a little bit more effort, since it's required to incorporate http-proxy package for this operation. Similarly to Webpack Dev Server, target
parameter defines destination of your redirection. The other parameter, ignorePath
, is set to false
by default. If you don't override it, /api
will be added to the end of your request's URL. Again, it might be what you want in some cases.
const app = express();
const apiProxy = httpProxy.createProxyServer();
// ...
app.get('/api', (request, response) => {
apiProxy.web(request, response, {
target: 'http://localhost:3001/get-secret-data',
ignorePath: true,
});
});
(content of the whole script available here)